Figma Plugin — JS Runner
If you’ve ever worked on Figma plugins, you know the rinse and repeat time suck: you write code, reload the plugin, and repeat—over and over. And over. And over. I wanted a better way. So, I built a Figma plugin that allows me to paste JavaScript directly into a popup, hit “Run,” and instantly execute it—no need to create a new plugin or reload every time.
This is a walkthrough of how I built it, so if you're not into nerd stuff this isn't the article for you.
This is part “plugin sandbox” for testing ideas faster, but also as an example of how to work with the Figma Plugin API faster for instances where you might want a one-off process. As I've blended AI with development I've found myself needing this more and more. Quite literally getting a step closer to the point where I can just tell ChatGPT what I want to do, copy the code, and paste it into this sandbox. Use it as you may.
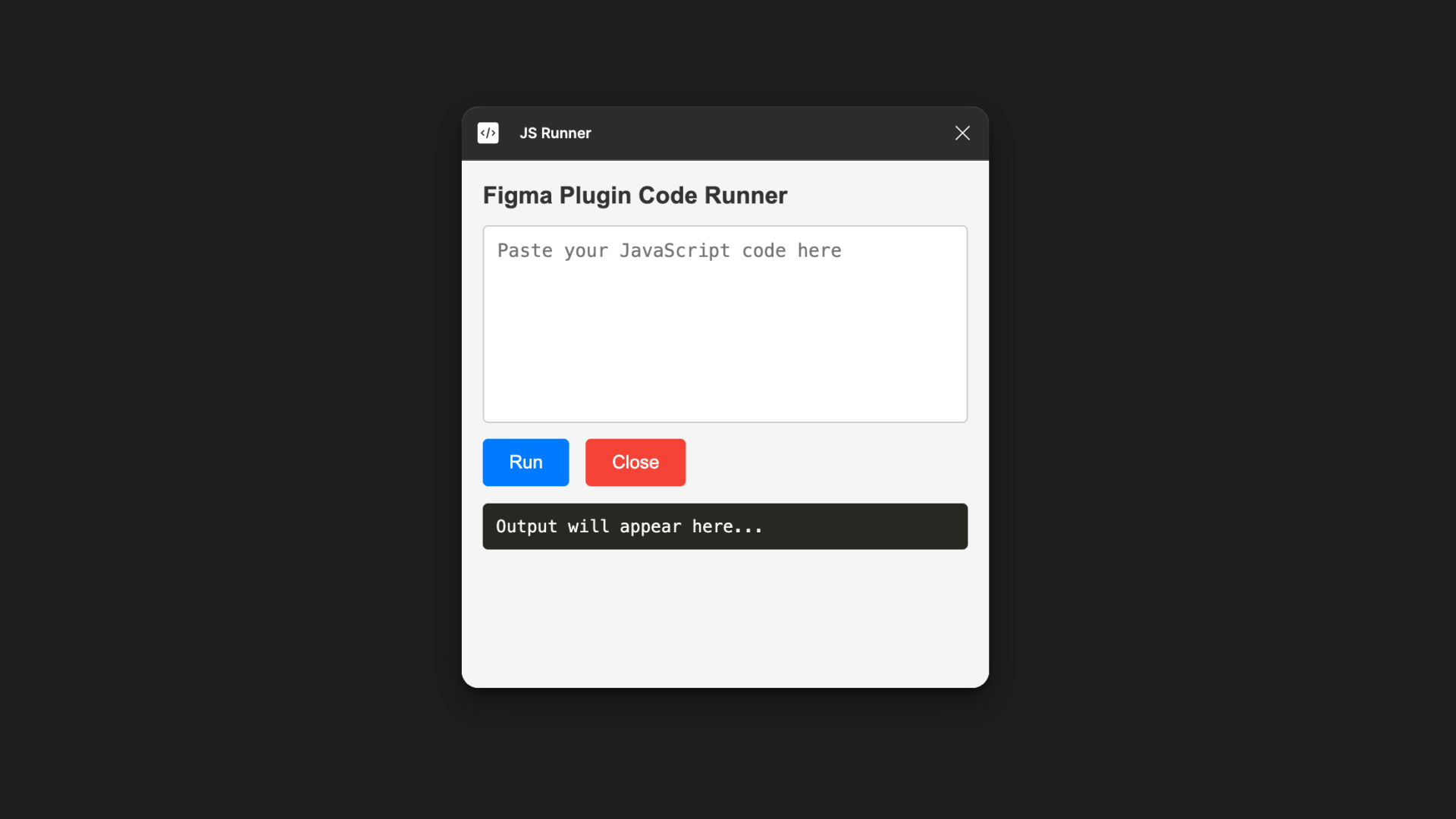
The Problem: Why Build This?
Developing plugins in Figma often means lots of iteration. But Figma doesn’t have a built-in way to execute arbitrary JavaScript during development, and setting up a plugin every time feels tedious for quick tests.
I wanted:
- A way to quickly test small pieces of code.
- Immediate feedback without reloading the plugin.
- A simple interface that wouldn’t distract me from the task at hand.
The Solution: A Code Runner Plugin
The plugin I built has a straightforward UI:
- A text area for pasting JavaScript.
- A “Run” button to execute the code.
- An output console for results or errors.
- A “Close” button to exit the plugin.
Building the Plugin
1. The Manifest File
Every Figma plugin starts with a manifest.json. Here’s the one I used:
{
"name": "Code Runner",
"id": "code-runner",
"api": "1.0.0",
"main": "code-runner.js",
"ui": "ui.html"
}
This tells Figma to load a backend script (code-runner.js) and a frontend interface (ui.html).
2. The Backend Script (code-runner.js)
The backend listens for messages from the UI and executes the JavaScript code you paste. It uses new Function() to isolate the execution (and catch errors).
Here’s the script:
figma.showUI(__html__, { width: 400, height: 400 });
figma.ui.onmessage = (msg) => {
if (msg.type === 'runCode') {
try {
const result = new Function(msg.code)(); // Run the code
figma.ui.postMessage({ type: 'output', result: result || 'Code executed successfully' });
} catch (error) {
figma.ui.postMessage({ type: 'output', result: `Error: ${error.message}` });
}
} else if (msg.type === 'closePlugin') {
figma.closePlugin();
}
};
3. The User Interface (ui.html)
The UI is where you paste your JavaScript and hit “Run.” I styled it to be simple but modern using CSS. Here’s the code:
<!DOCTYPE html>
<html>
<head>
<style>
body {
font-family: Arial, sans-serif;
padding: 16px;
background-color: #f5f5f5;
color: #333;
}
textarea {
width: 100%;
height: 150px;
margin-bottom: 12px;
padding: 10px;
border: 1px solid #ccc;
border-radius: 4px;
}
button {
padding: 10px 20px;
margin-right: 8px;
border: none;
border-radius: 4px;
cursor: pointer;
font-size: 14px;
}
button#runButton {
background-color: #007bff;
color: white;
}
button#closeButton {
background-color: #f44336;
color: white;
}
pre {
background: #272822;
color: #f8f8f2;
padding: 10px;
border-radius: 4px;
margin-top: 12px;
}
</style>
</head>
<body>
<h1>Code Runner</h1>
<textarea id="codeInput" placeholder="Paste your JavaScript code here"></textarea>
<button id="runButton">Run</button>
<button id="closeButton">Close</button>
<pre id="output">Output will appear here...</pre>
<script>
document.getElementById('runButton').addEventListener('click', () => {
const code = document.getElementById('codeInput').value;
parent.postMessage({ pluginMessage: { type: 'runCode', code } }, '*');
});
document.getElementById('closeButton').addEventListener('click', () => {
parent.postMessage({ pluginMessage: { type: 'closePlugin' } }, '*');
});
window.onmessage = (event) => {
const msg = event.data.pluginMessage;
if (msg.type === 'output') {
document.getElementById('output').innerText = msg.result;
}
};
</script>
</body>
</html>
That's it!
If you’re tinkering with Figma plugins, I highly recommend building a “sandbox” like this. It’s a time-saver, a great learning exercise, and a surprisingly fun project.
Let me know if you try building it—or if you have any ideas for improving it!